How to Create an Abstract Class in PHP
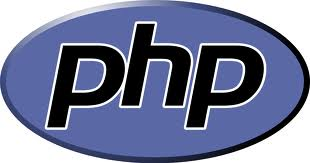
In this article, we show how to create an abstract class in PHP.
An abstract class is a class that which an object cannot be instantiated. Instead the class serves as a blueprint for children classes of this abstract class. In a PHP abstract class, you must define at least one abstract method. Each child of this abstract class then can implement the method or methods specified in the abstract class.
In other words, all an abstract class does is serve as a future blueprint for children classes in terms that it lays out the methods the children classes should implement.
The abstract class normally doesn't implement the method themselves. Normally the methods are empty. The name of the method is just declared so that it can be defined for future children classes.
So the parent class is abstract. The children classes are tangible.
To make a class abstract in PHP, all you have to do is put the keyword abstract before the keyword class. This makes the class an abstract class.
As stated, the methods of the abstract class must be labeled abstract. This is done by putting the keyword abstract in the method declaration.
So, as an example, below, we created an abstract class called shapes.
So we created an abstract class called shapes.
Inside of it, we created an abstract public function called calculateArea().
When you think about this, it makes sense. Shape can be seen as a generic or abstract concept. A shape is can be one of many things. It isn't specific.
So a shape object really cannot be instantiated.
However, if you create a child class in which you specify the shape, you can have child classes such as circle, triangle, square, rectangle, etc, which are definite shapes.
So a shape is abstract. A circle, rectangle, triangle, is tangible. It's a definite shape.
You cannot calculate the area of a shape, since it's generic.
However, you can calculate the area of a circle, rectangle, triangle, since it's tangible.
So this is the methodology behind abstract classes.
Now, that you understand the concept behind, there are a number of rules for using abstract classes in PHP.
As stated previously, abstract classes must contain at least one method that is also marked abstract. These methods are called abstract methods.
If a class contains an abstract method, the class must also be declared abstract. However, abstract classes can contain non-abstract methods.
Abstract methods are not implemented in the abstract class. Instead the methods are implemented in a child class that extends the abstract parent.
If a subclass fails to implements all the abstract methods in the parent class, then it itself is abstract and another class must be created to further subclass the child and implement the remaining methods.
Abstract methods cannot be defined as private, because they need to be inherited.
Abstract methods also cannot be defined as final, because they need to be overridden.
Lastly, when you specify the abstract method in the parent class, the exact same method with the exact same parameters must be used in all the children classes. Meaning you cannot
have a method that accepts different parameters in any of the child classes. It must be the exact methods that receives the same exact arguments, or else an error will be output.
So just to give a complete example of abstract code, see the following code below.
Complete Code of an Abstract Class
You can see how we create an abstract class called shapes.
This abstract class has an abstract method called calculateArea(). This method will give us the formula for the shapes we specify. Each child class must implement this calculateArea() method.
So we create our first child class under the abstract class named circle. In this class, we create a public function called calculateArea(). This calculateArea() function when called will show the formula for calculating the area of a circle.
We then create another child class of the abstract shapes class named square. In this class, we create a public function calculateArea(). This funtion when called will show the formula for calculating the area of a square.
We then create objects of each of the classes and call the methods.
This leads to us getting the following output shown below.
Actual PHP Output
You calculate the area of a circle by the formula, A= πr2
You calculate the area of a square by the formula, A= (length)2
So you can see how the abstract class provides the abstract foundation. And then we create child classes that actually implement the methods.
So this is a quick run-down of abstract classes, how it can be created, and the rules that apply for them in PHP.
Related Resources
How to Create a Final Method in PHP