How to Count the Number of Objects in a Class in PHP
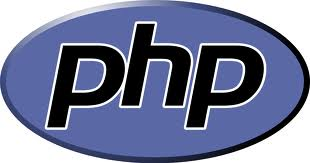
In this article, we go over how to count the number of objects in a class in PHP.
Every time we instantiate (create) a new object, we can keep track of this through a static property. Thus, we can count the number of objects instantiated for a given class.
As far as I know, PHP does not have a built-in function that counts the number of objects in a class, so we simply create a custom one and it's very easy to do.
We create a static property (since it isn't tied to an object but to the class) and we put inside of the constructor. Since the constructor is called every time that an object is instantiated, we add increment the static property keeping count by 1 each time an object is instantiated, we can keep track of the number of objects in a class.
So in the PHP code below, we have created a program that counts the number of objects created for a class in PHP.
So in this code, we create a class named cars.
We declare a public static property named $count and we set it equal to 0. This variable will be used to count the number of objects created in a class.
We then create the constructor for the class. Since this is a class that represents cars, each object created is a car object (represents a car). We want to pass into the car objects the type and year of the car.
So in the constructor, we pass in the arguments $type and $year, representing the type and year of the car.
We then use the $this keyword to assign the value of the type and year to the $type and $year variables.
We then use the increment operator to increment the cars::$count property.
Because the constructor is called each time an object is created, we can use this fact to keep track of how many objects are created. Initially, the cars::$count variable is 0. However, with each object that is instantiated, the count goes up by 1. Thus, we're able to know how many objects have been created.
Since we've created 5 cars, at the end of this program, you'll see that the program says there are 5 objects created.
Actual PHP Output
The number of objects in the class is 5
Keeping Count of the Number of Objects If Any Are Deleted
You could make this program a little more elaborate.
For example, what if an object is destroyed? What if we delete one or more of the objects after they are created?
In that case, you would also have to make a destructor function for the class. When an object is destroyed (if you unset an object) then you place in the destructor function, a decrement operator for the cars::$count variable. So if an object is destroyed, the count goes down by 1. This way, you can keep track of how many objects currently exist for a class.
So below is the code that keeps track of all currently existing objects in a class. If an object is created, the count goes up by 1. If an object is destroyed, the count goes
down by 1.
So all the code is mostly the same except now we have a class destructor and we intentionally delete an object, $car4. Inside of the destructor, we have the cars::$count variable decrementing by 1 each time the destructor is called. And the destructor function is called when we delete an object through the PHP unset() function. So whenever we delete an object, the count goes down by 1.
So now when we run our code, now that we've deleted one of the objects, the count will be 4.
So if I run the PHP code above, we get the following output below.
Actual PHP Output
The number of objects in the class is 4
So using a static variable is a very effective and easy way to keep track of the number of objects in a class.
Because a static variable isn't tied to an object and is instead tied to a class, it is independent of any object in the class. Therefore, it has an outsider view, so to speak, and can keep track of all objects in a class.
So this is how you can keep track of the number of objects in a class in PHP.