How to Generate a Random Password Using PHP
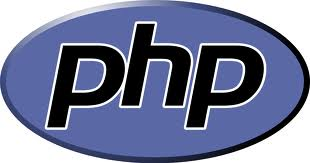
In this article, we show how to generate a random password using PHP.
Refresh this page and see the random password change each time.
Random Password: ps,H3viuqz
Many sites use random passwords.
For example, say you go to a website. This website needs you to login in to your account. Say you don't remember your password and can't log into your account. You then click the link "Forgot Password". This page may require you to enter in some additional information so that it can verify that you are in fact the account owner. Once you've done this and submitted the information, it may email you a temporary password. You then go back to the login page, type in the temporary password, are granted access into your account, where you can then change the password to any password you want.
This is done for many, many sites on the web.
So maybe you want to implement a system like this on your website.
So now we can go over how this can be done with PHP code.
So, for the random password generator I've decided to create, I made it so that the random password is a 10-character string. You can make it however many characters you want.
Obviously the more characters you have, the harder it is to guess a temporary password. This, in turn, makes the account less vulnerable to being hacked into, in case someone is trying to do so.
However, you also don't want to make it so long that it's like 30 characters. Because it's really unnecessary.
For this password, we'll have a variety of characters in the string including special symbols and numbers. This makes the password more difficult to guess and, thus, strengthens security.
So the PHP to create this random password generator is shown below.
So now let's go over all the code required to make this random password generator, in case you want to be able to modify it.
So the first thing that we specify in the code is the password length. In this code, we chose a length of 10 characters for the password generated. You can change it to any amount. I mean, you do want it to be a reasonable amount, though. You don't want a password that's 50 characters in length long. That's unnecessary. So anywhere from 8 to 12 is reasonable. But of course you're free to do what you want.
After we specify the length of the password, we created a list and special symbols and store it in the $symbols variable. You can add whatever special symbols you want added. This is totally a free list. You can add any characters you may want to appear in the random password.
We then create a variable named $symbolscount. This counts the number of special symbols in the $symbols variable we created before.
We then create a variable named $randomposition. We use the mt_rand() function to pick a random position that runs the length of the $symbols variable. The $symbols variable runs from an index of 0 to $symbolscount - 1. The mt_rand() function is a function that chooses a random value from a list of values. Since we want it to pick any position in the list of the symbols (with the list starting from 0 and ending at $symbolscount - 1), the full line of code is, $randomposition= mt_rand(0, $symbolscount - 1);
Next, we actually begin creating the password. We use the substr() function to take a part of the $symbols variable. It takes a single random character from the $symbols variable. We place this single random character in the $password variable.
We then take a random character from the ASCII values of 48-57 and append it to the $password variable. The ASCII values of 48-57 pertain to the values of 0-9. A random character is selected from 0 to 9 and appended to the $password variable.
We then take a random character from the ASCII values of 65-90 and append it to the $password variable. The ASCII values of 65-90 pertain to the values of A-Z (uppercase characters). A random uppercase alphabet character is selected from A to Z and appended to the $password variable.
We then have a while statement. While the length of the password is less than the length that we want it to be, we append a lowercase alphabet character to the password. The ASCII values of 97 to 122 pertain to a-z, the lowercase alphabet characters. As long as the password is less than the length we specify and want it to be, lowercase alphabet characters are appended to the password string. Once the length of the password is what we specified, then we come out of the while loop.
After this, we use the PHP str_shuffle() function to shuffle the characters in the string in any type of order.
We then output the password.
Note that there are several variations that can be done. For example, we don't simply have to add one special symbol. We can add 2 instead. The modification to the code necessary to do so can be found at the following link: Code to add 2 special symbols to the password of the random password generator.
If you want to add a second number to the random password string, you would just duplicate the exactly the line of code, $password .= chr(mt_rand(48,57)); With 2 of these lines in the code, this adds 2 numbers to the random password string instead of just one.
So now that you know these modifications, you can generate any random password you want of any length.