How to Serialize an Object or Array in PHP
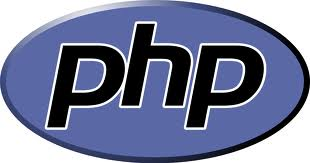
In this article, we show how to serialize an object or array in PHP.
By serializing data, an object or an array, we mean we convert the data to a plain text format.
So the an object gets converted into a plain text string.
And an array gets converted into a plain text string.
Serializing data is useful for many reasons.
It is useful mostly for when you are passing data outside of PHP.
This is especially true for complex data structures, such as arrays and objects. Other types of simple data structures such as integers or strings can work fluidly with other languages and applications as is.
But other languages can't understand native PHP code when it comes to complex data structures, so serializing the data (converting the data to a plain text string) allows the data to be able to be used in other applications outside of PHP. So if you are putting data into a MySQL database, for instance, you may serialize the data before transmitting it to the database. For example, you can't just put a PHP object in a MySQL database. It's pure PHP code. It won't understand it. Thus, you serialize the object and then you can transmit to the database. The same is true for an array.
Even though there are other ways of doing it, serializing the data is one of the options so that the PHP code can be transmitted to other languages and applications.
In this way, you can view serializing data as making the data platform or language neutral.
Practically every language can understand a plain text string, so this data can work with other applications.
Serializing an Object
So we'll now show how to serialize an object in PHP.
This converts the object to a plain text string.
Below is PHP code that serializes an object in PHP and outputs the serialized data so that you can see its form.
The PHP output of this code is shown below.
Actual PHP Output
This is the serialized object: 'O:4:"cars":0:{}'
So you can see the output above.
We'll explain the code of it now below.
So we create a class called cars.
Right underneath this class we instantiate an object of the class, $car1. This is an object of the cars class.
We then serialize this $car1 object.
After this, we then echo out the value of the serialized car object.
Now having undergone serialization, the $car1 object is now a string and can be used easily outside of PHP.
If you ever want to convert it back to an object, its native PHP code, then you would use the PHP unserialize() function. This converts it back to its native PHP object.
This is shown below.
So you can serialize the object for it to be able to work with applications outside of PHP and unserialize it to
convert it back to native PHP code.
Serializing an Array
In the same way that we serialized an object, we can serialize an array.
An array is a complex structure that may need to be serialized to work with outside applications of PHP.
The PHP code below serializes an array and shows the output.
This PHP code yields the following shown below.
Actual PHP Output
This is the serialized array: 'a:4:{i:0;s:4:"blue";i:1;s:6:"orange";i:2;s:5:"black";i:3;s:5:"green";}'
So it's really the same principle, just now with arrays.
Again, just like with objects, you can unserialize the array and get its back to its native PHP code.
And this is all that is required to serialize data in PHP.
Related Resources
How to Unserialize an Object or Array in PHP