How to Create Your Own File Transfer Protocol (FTP) Upload For Your Website Using PHP
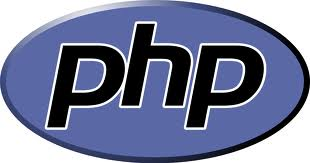
This is a simple, functional File Transfer Protocol that allows file uploads (one-way communication)
This particular FTP being demonstrated below is restricted to protect this site
However the code shown below does not contain these restrictions
Uploads can only be done to the Uploads Folder or the Documents Folder for demonstration purposes
The file uploaded cannot be a PHP, CSS, ASP, JS, or XML file to protect the site
The file cannot be an index file to protect the site
The password of the FTP below is abc
In this article, we demonstrate how to build a simple File Transfer Protocol (FTP) Upload using PHP.
It's a simple FTP that allows you to upload any type of file to any directory (folder) on your website.
It's simple because it's one-way communication. It doesn't allow you to see a directory of files on your site. However, it allows you to upload any new document to any folder on your site.
So it's not a full 2-way FTP that allows you to obtain files already uploaded to your site, but it allows full uploading to your site.
How it works is through PHP file uploading. We create an upload form using HTML. And then we can upload files to a server using PHP code. Using PHP code, we can transfer this file to any directory on our website.
And this is all that is needed to create a one-way file transfer protocol system.
It's almost completely necessary to add a password text box to a FTP because you want to protect your site. You have to have some security measure in place so that no one can just find your FTP on your website and upload anything that they want on it. It is very smart to add a password option. You need some security measure to protect your website. Some people may find it annoying to have to put in a password every time they upload a file to their website, but it's worth someone damaging your site. Just imagine, you create a website and you have pages uploaded to your site. Now someone comes along and uploads the same files you have, overwriting those files, and now you've lost all your pages on your website. A hacker could even upload an index file and erase your homepage. This is why security is so important. One good strong password protects heavily against this. However, if you decide that is just too annoying to have to put in a password each time that you upload a file to your site, then you can see the following code which creates an FTP that doesn't require a password at the following link: Code for File Transfer Protocol that Requires No Password . You would have to put this FTP in some archaic name on your homepage so that it isn't easily found.
This FTP needs to get published to your root folder on your website (the home page folder). This needs to be done so that you could reach all the directories on your website. How the PHP move_uploaded_file() function works is it takes the current directory you're at and you can upload a file to that directory and further directories in that pathway. So publishing the FTP to your root directory (which contains the home page) allows you to reach any directory on your website. So this is the best way to do it.
Other than this, the FTP contains a textbox that allows you to specify which directory (or folder) you want to upload (or publish) the document to. If you keep this text box blank, it uploads the document to the root directory of your site. So if your site is www.example.com and the file you uploaded is monkey.jpg, the image file can be found at www.example.com/monkey.jpg.
If you type in images to the Upload textbox, the monkey.jpg image will be found at www.example.com/images/monkey.jpg. Also know that you can enter /images, images/, or images, and all will produce the same result. This is because the code we have running corrects either 3 of them so that they all work. So you don't have to worry about the same technical of putting in the directory. You can simply just put the name of the directory and it will work out.
One last example, if you type in Documents and you have a folder named Documents and you upload the file, bills.pdf, this will appear at www.example.com/Documents/bills.pdf.
So you pretty much get the idea of how this works.
All you have to do is copy all 3 codes found below and save it as a PHP page and publish to the root directory of your website. Before you do that, change the password to 'abc' to something much harder. Then you will be able to upload any document to any directory on your website.
So this is a very easy, simple, and highly effective way to upload files to your website.
One question you may have, though, is why use this? Why use it? If you have a good hosting company for your website or you have Filezilla, an excellent FTP, why would you need an FTP on your own website?
And there are many reasons why.
First of all, usually for hosting companies, even godaddy, you have to login to your login account. Then you have to go to your hosting plan, find the file transfer protocol on the website before you can transfer files. This is still a good amount of effort. If you have your own FTP on your website, all you need is just type in the URL and you're there.
Now with an FTP like Filezilla, the FTP has to be downloaded to the computer in order to be used. Filezilla, as far as I know, is not available on the cloud. Therefore, it has to be downloaded to your computer. This is no problem if you have your own laptop available that has the FTP downloaded on it. But this is not always the case. Imagine you're at work and you have spare time and you want to publish a file to your website. Most computers at work require administrator permission in order to download any type of software on it. So most of the times this is not a possibility. This is the case the majority of the times for any type of public computers such as at libraries or schools. IT adminstrators ban the download of software. Therefore, the only option to upload documents to your website is through your web hosting company website. This is fine, but it's not as quick and efficient as creating your own FTP and placing it directly on your site. Placing an FTP on your site is the fastest, most efficient way to upload and publish new pages to your site. So if you have files available on your flash drive, dropbox, or email that you would like to publish right away to your site, you can do it from your own website.
So below we go over how to do this.
HTML Code
So below we will start with the HTML code needed to create the file upload form.
So the HTML shown above is very basic.
We create a form, a file upload form. We set the action equal to #upload. This allows us the form, once submitted, to jump to where we have <a name="upload><a/>. We set the method equal to POST, because it's a large amount of data and we don't want the data appended to a URL. We want the data in an actual location, which is where we're uploading the image file to. The enctype statement is unique and needed for file uploads. It must be present.
The next line creates a file upload button. We name it "file".
The next line creates a submit button. Since it's an upload button, we name it "Upload".
We then end the form.
This is all the HTML code needed. The HTML simply creates the upload form.
The PHP code shown below is what actually adds functionality to the upload form so that it actually performs
an upload.
PHP Code
There are 2 PHP blocks of code to make this image upload work.
The first and the biggest block is shown below.
So this is obviously a big block of code. We'll explain it all now below.
The $name variable stores the name of the file being uploaded. This includes the name of the file plus its extension. So if upload a file named mortage and its of the format pdf, the $name variable stores mortgage.pdf.
The $tmp_name stores the temporary name of the file. When we first upload the file, it isn't carried over directly to the final destination, which is the folder we want it transferred to. Instead, it gets a temporary. We then have to use the function, move_uploaded_file() to transfer the file using the temporary name to its final destination folder. So, again, the variable $tmp_name is very important for uploading the file.
The $submitbutton variable stores whether the submit button has been clicked or not.
The variable $where_to_upload takes the input entered into the place where a user wants to upload the document to and stores it. Just in case there is any unnecessary, unintentional space entered in by the user, the next line erases any whitespace.
The $password1 variable takes the input that a user has entered into the password text box and stores it.
The variable $password2 is where you set the password in your code. You can set it to any password you want. Obviously, you want to choose a somewhat difficult password to figure out so that your website is more secure. You definitely want to change the password from 'abc' to something else.
The variable $length stores the length of the $name variable, which is the variable that stores the name and file extension of the file being uploaded. The reason we find out the length of the string is because we want to know how long it is, so that we know the position of the last character. The last character will be at the string length minus one. The reason we do this is because we want to know whether the last character is "/" or not. If it is not, we append "/" to the string. If it is, we leave it alone.
The variable $first represents the first character in the string. We want to see whether this character is "/" or not. If it is, we remove it. If it is not, we leave it alone.
We do this so that the pathway can be completely correct.
Now we go into the heart of the code.
We use an if statement to see if the $name variable has been set. This means that the submit button has been clicked, in other words.
If the $name variable is empty, then the user hasn't specified any file in the upload. Therefore, we output the statement, "Please choose a file".
If the $name variable isn't empty and the password entered by the user matches the password you set in the PHP code, then the file is uploaded using the temporary name to place you specified in the where to upload textbox.
Else, if the password entered is incorrect, we output the statement, "The password entered is incorrect. The file has not been uploaded."
This completes the first block of PHP code.
The second block of PHP code is shown below.
So this block of PHP code is much smaller.
If the $name isn't empty and the password entered is correct, the statement output is, "The file you uploaded is shown below." The link to the file is then shown below.
The one annoyance that can be used with the FTP we created is that each time you have to enter in your password when uploading a file, while with your hosting company or filezilla, once you've logged in, you don't have to reenter your password with each file upload. However, this is is a very small annoyance and be none at all, especially since most browswers today have have an password autosave option, so that it stores the password so that once it is entered it doesn't have be to reentered.
So this is all that is required to build an FTP that allows file uploads.
Related Resources
How to Upload Images to a Website Using PHP
How to Upload Videos to a Website Using PHP
How to Upload Files to a Website Using PHP
How to Redirect to Another URL with PHP
How to Create a Search Engine Using PHP
How to Insert Images into a MySQL Database Using PHP
How to Create a Searchable Database Using MySQL and PHP
How to Search a MySQL Table For Any Word or Phrase Using PHP
How to Create a Send Email Form Using PHP and MySQL