PIC Programming- ADRESL & ADRESH
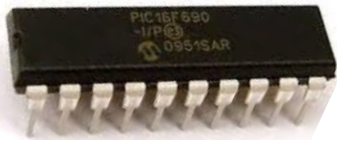
The ADRESL and ADRESH registers are 2 registers used to store the end result of an analog-to-digital conversion (ADC).
These registers end up storing the analog value reading.
The reason that 2 registers are used in the ADC process is because PIC microcontrollers typically perform 10-bit ADC conversions (or greater). A single register is only 8 bits wide. So it cannot hold a 10-bit value. Therefore, an ADC conversion must go into 2 registers. These 2 registers are ADRESL and ADRESH.
ADRESH and ADRESL really stand for A/D Result Registers High and Low Byte.
ADRESL is an 8-bit register. It holds 8 bits. So by itself, it can only count up to 256 (28=256). Therefore, the ADRESH register is needed to count up to the full 10 bits (210= 1024).
How it works is that the analog result will be.
RESULT= ADRESL + (ADRESH * 256)
The low byte ADRESL counts up to 256 and overflows. The high byte ADRESH is incremented every time ADRESL overflows. ADRESH is counting how many 256s there have been.
Since ADRESL is only 8 bits, it can count only up to 28, which is 256. After that, ADRESH updates to count how many 256s t there have been. Both together can tell us of an ADC value which can go up to 1024 in 10-bit ADCs (210= 1024 value).
To see how this works, let's go over a full coding example.
Complete Coding Example with ADRESL and ADRESH
The following code takes an analog reading from a potentiometer. The potentiometer is connected to analog pin AN0.
Below is the code:
#include <p18f1220.h>
#pragma config WDT=OFF, OSC=INTIO2, PWRT=ON, LVP=OFF, MCLRE=OFF
#include <delays.h>
int analog_reading;
void main(void)
{
//SET UP
ADCON1= 0b01111110;
TRISA= 0b11111111;
TRISB= 0b00000000;
PORTB= 0b00000000;
ADCON0bits.ADON=1;
ADCON2= 0b10000000;
while (1)
{
ADCON0bits.GO_DONE=1; //do A/D measurement
while(ADCON0bits.GO_DONE==1);
analog_reading= ADRESL + (ADRESH *256);
}
}
Related Resources
How to Program a PIC Microcontroller