How to Use Sessions to Track User Data Using PHP
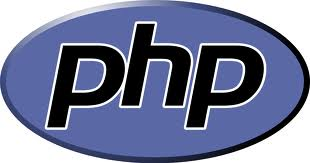
In this article, we show how to use sessions to track user data using PHP.
This is actually a powerful concept because sessions data can stored on the web server. Therefore, if a user clicks and goes to another page, the server still has the information about the user.
Many sites use this concept of sessions. Pretty much any site that has a login system where a user logs in will use sessions. Once a user is logged in, a session is begun. If the user goes to another page or even gets off the site to another and comes back, their data is still available, so they can pick up right where they left off.
Shopping sites use this all the time. You log into your account and no matter what page you go to on the site, you're still logged into your account and the website knows who you are.
So how do sessions work and how can we implement them on a website with PHP?
Form To Track You Through Sessions
Before we go get into the code for this tutorial, enter your first name into the text box above.
This form will illustrate sessions for you.
You will see how you go from page to page, yet each page knows your first name. This is done through sessions.
We will now go over how to do this below in code.
HTML Code
So let's say just like above we have an HTML form that gets data from a user, such as above with a text box asking for the user's first name.
The HTML code for this is shown below.
The <form></form> tags create an HTML form.
We set the action attribute of the form to userpage.php. This is because this is the PHP page we want to transfer the user to when the user clicks on the Submit button.
The method is set to POST because we're posting it to the actual page, not the URL, which is what the GET method does.
We then have a standard HTML text box that asks for the user's name.
This is followed by a submit button, which allows a form to be submitted.
PHP Code
So now we go to the first PHP code that is used once the user submits the form.
The contents of the PHP file, userpage.php, is shown below.
So the first thing that we do is call the function, session_start().
Session_start() is one of the crucial of sessions. Without it, sessions could not work.
In order for you to start a session that is able to track a user or user data, you must start a session. This is what the session_start() function does.
This code must exist before any other code on a page, or else it will not work. Therefore, when you use the session_start() function, do not include any other code before this of any kind, including HTML code. You will get the error, "Headers already sent". You must put this first in your code. If you still get the error, "Headers already sent" and you are not including any white space or code of any kind before the session_start() function, then save your PHP file with the ANSI encoding. More than likely, your PHP file is saved either with Unicode, Unicode Big Endian, or UTF-8 encoding. These type of encodings add invisible characters before that causes PHP to throw errors.
So after this session_start function, we retrieve the data that the user entered into the form. Since the name of the HTML text box was name_entered, we use the superglobal array $_POST to retrieve the data entered into the this text box; we assign the value of this data to the variable $name.
The next line of code is very important. We create a sessions variable called name that is set equal to what the user has entered into the text box. This way, $_SESSION['name'], is equal to the name that the user has entered. This is how we will track the user or the user's data from page to page.
Because session variables are saved on the server, even if a user goes to another page, this sessions variable is still stored. Therefore, we can track users from page to page to page. This, again, is how many sites that have users logged in allow a user to see they are still logged in even when going from different page to different page.
So on this userpage.php file, we transfer the user to another page to show that we can track the user (the user's data) through session variables.
This page is anotheruserpage.php, which we show the contents of below.
The biggest thing to note about this page is it has another session_start() function.
In fact, every page that tracks a user through sessions must have the session_start() function at the top of the PHP code.
The session_start() function is used for when a session is first begun and to continue a current session for a user. It might seem a little weird to have a session called session_start when you're continuing an already started session, but look at it like this, it starts a session for each page, so that the session is continued on other pages. That's the way I see it, at least.
So, again, for each page that tracks user data through sessions, there must be a session_start() function on that page. So if you have a very interactive site where you want a user to be logged in no matter what page they are visiting on your website, that means you will have a session_start function on every page on your website.
So after this session_start() function, we output a statement that we still know who the user is even though this is another completely separate page. And we know this because of the session variable, $_SESSION['name'], that we created. Once a session variable is created, which we did on the userpage.php file, and we use the session_start() function on each page after that, the server still has the value stored in this session variable. So if we call it, it still has the value. So whatever you entered into the HTML form is still contained even after now 2 PHP files.
After this page, we demonstrate one last page tracking the user data through sessions. Why not?
The page we transfer the user to now is lastuserpage.php.
The contents of the lastuserpage.php file is shown below.
So, again, as explained, we must include the session_start() function. This is needed on every PHP page that you want to track session data, either to create a session or maintain a current session.
We output the user's name (stored in the $_SESSION['name'] variable) and say this is just one last page to show you the
session data transfers over.
And this is really all that is required of the basics of working with sessions.
If you want to end a session, a session normally ends when a user closes the browser or even sometimes when a user goes to a website. But if you want to definitively end a session with PHP, then you can use the session_destroy() function. This terminates a user's session so that the user is no longer being tracked.
In this article, we just showed one example of a session variable but you can store as many session variables as you want.
In our form, we just tracked the user's first name. However, we can track one of hundreds of variables if we want, including last name, email address, phone number, etc.
We can track anything. This can be data that is entered into an HTML form, such as you did. Or you can have a user log in on a site
and have data from past history such as the long form that have to complete when they're initial registering on the site or their shopping history based on past purchases.
You may have this data stored in a database and then pull it from the database and store it in session variables for the duration of the session. So there's many dynamic
ways of doing it but sessions is a powerful programming concept to learn and many sites use it to track users from page to page.
Related Resources
How to Create a New Directory Using PHP
How to Upload Files to Your Server Using Your Own Website
How to Restrict the Size of a File Upload with PHP
How to Create Your Own File Transfer Protocol (FTP) For Your Website Using PHP
How to Create a Register and Login Page Using PHP
How to List All Files in a Directory using PHP
How to List All the Directories of a Website Using PHP
How to List All Files of the Home Directory and Its Subdirectories of a Website Using PHP
How to Read the Contents of a File Using PHP
How to Write Contents to a File Using PHP
How to Copy a File Using PHP
How to Rename a File Using PHP
How to Delete a File Using PHP