Arduino Motion Detector Circuit
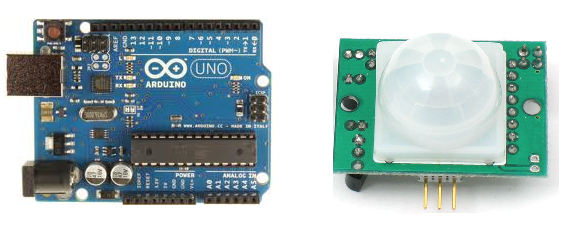
In this project, we will go over how to build a motion detector circuit with an arduino.
With a PIR motion sensor integrated with an arduino, we can detect movement and program the arduino to do something once motion is detected.
For example, once the motion sensor picks up motion, we can program the arduino to turn on an LED, turn on a motor so that it spins, sound a buzzer off, etc.
With the arduino integrated into the circuit, through software, we have total control of what we want to do once motion is detected.
In this circuit we are doing here, for simplicity, we will simply turn on a buzzer once the motion sensor detects motion.
However, like explained before, you can customize the circuit to do anything.
Components Needed
- Arduino Board
- PIR motion sensor
- 6V Buzzer
- USB with type A and B connectors
The main electronic component we will use that allows us to pick up this detection is the PIR motion sensor. The PIR motion sensor is a sensor which detects movement through picking up infrared radiation. Being that a person emits infrared radiation, the detector is able to detect this and react, according to the how the circuit is designed to react. The sensor can also pick up the movement of inanimate objects as well, such a rolling ball, because as those objects move, friction acts on them, generating heat. This heat emits infrared radiation, which the PIR sensors may be able to detect if great enough.
This motion sensor can be bought at various online retailers. Probably the most reasonable price to get it is from amazon.com at the following link: Amazon- PIR Motion Sensor.
The PIR motion sensor is a 3-pin device. Below is the pinout of this device:

Pin 1 is the pin which receives the positive DC voltage. The PIR motion sensor needs between 5V-9VDC of power for operation. In our case, we will use about 6V of power. This can be obtained from switching a DC power supply to 6V or using 4 'AA' batteries connected in series. We will then feed this voltage into pin 1 of the PIR module.
Pin 3 is the negative DC voltage or ground pin of the device. We connect the negative terminal of the power source to this pin, for a return path.
Pin 2 is the Output pin of the PIR module. This is where the output of the PIR will leave from. When motion is detected by the PIR, its output will go high to 3V. When no motion is detected, its output low and it gives off practically no voltage. When high you can see then how it can power a load, such as an LED to light. This way we can know when it has detected motion or not.
This sensor has a sensitivity range up to 20 feet (6 meters) and a 110° x 70° detection range, making it a wide lens detection sensor. This means it can measure 110° vertically (from top to bottom) and 70° horizontally (from left to right). The best way to check its sensitivity is when the circuit is built, try moving around through all of its angles. See at which angles it can detect your movement and at which angles it is not able to detect your movement, meaning your out of its angle scope. A lot of it is trial and error and experimenting. Once you know where it can and cannot detect, you can place it in an optimal place where it can detect in areas where you want it to.
Besides this sensor, we need a buzzer which we will light when motion is detected.
You can use any arduino board. You will need a USB with a type A connector at one end and a type B connector at the other.
The type A side connects to the computer you will be using and the type B plugs into the arduino USB slot. This way, we can program
and upload software for the arduino board to follow.
Arduino Motion Detector Circuit
The arduino motion detector circuit we will build is shown below:

The schematic diagram of this circuit is:
Pin 1 of the motion sensor connects to the 5V DC voltage terminal of the arduino. Pin 3 connects to the ground (GND) pin of the arduino. Pin 2 connects to the digital pin D3.
With this connection, pins 1 and 3 are powered with 5V by the arduino, so it is through these pin connections that the PIR motion sensor gets the 5V that it needs to power on and operate.
And it is through pin 2 that the arduino receives output from the motion sensor. When the motion detector does not detect any motion, the output is LOW and the arduino receives no voltage signal. When the sensor detects motion, the output is HIGH and the arduino receives a voltage signal, which can then trigger another device to turn on, such as a buzzer we will use for this circuit.
The buzzer we will power connects pin 12 and ground of the board. The anode connects to pin 12 and the cathode connects to ground.
Program for Arduino Motion Detector Circuit
After we connect the USB from the arduino to the computer, we are ready to write the code that the arduino board will need uploaded to it so that it knows to turn on the buzzer when motion is detected.
The following code, or sketch, will turn on the buzzer on pin 12 when the sensor detects motion:
const int buzzerPin= 12;
const int motionSensor= 2;
void setup(){
pinMode(buzzerPin, OUTPUT);
pinMode(motionSensor, INPUT);
}
void loop(){
int value= digitalRead(motionSensor);
if (value == HIGH)
{
digitalWrite(buzzerPin, HIGH);
delay(500);
digitalWrite(buzzerPin, LOW);
}
}
The first block of code chooses the pin for the buzzer, which is pin 12. The second line chooses the pin for the input pin, which represents the PIR sensor, pin 2.
The second block of code declares the buzzer as output and the output of the PIR motion sensor as input.
The third block of code reads the input value and assigns it to the integer value. It reads whether the input pin is HIGH or LOW. If it is HIGH, then
the motion sensor has detected motion. If it is low, the sensor has not detected any motion. If the value is
HIGH, it turns the LED on, signaling that motion has, in fact, been detected. The LED stays on for 500ms, or a half a second, and then goes back low.
You can modify this code so that the LED stays on for 1 minute after motion has been detected. You can modify it so that it stays on for 30 seconds. All this can
be done by changing the value given to the delay() function. 1 minute would be 60000ms. 30 seconds would be 30000ms. Customize the code to suit your circuit's needs.
To see how this circuit works in real life, see the video below.
Related Resources
Arduino LED Flasher Circuit
How to Drive a 7 Segment LED Display with an Arduno
How to Connect and Read a Keypad with an Arduino
How to Display Text on an HD44780 LCD Using an Arduino
How to Build a Hall Effect Sensor Circuit
How to Build a Vibration Detector Circuit